Author |
Message |
leu_raz

|
Posted: Wed Jan 05, 2005 9:14 pm Post subject: Poker Game |
|
|
Hello there compsci.ca team.
I am starting a Poker Game. I will work in Java(RTP....)
The thing is that I do no know how to make my program
'understand' when it's gonna have a Pair/Flush/etc.
May I hvae your suggestions please?
And basically how to do it without mouse,i know it's gonna be a crappy game in the end because the mouse really helps you out a lot.
Thank you very much
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
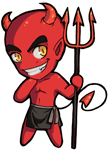
|
Posted: Wed Jan 05, 2005 9:45 pm Post subject: (No subject) |
|
|
the trick is to sort the cards in order by their face value. I don't remember if Java has any build in sorting algorythms, it shouldn't matter... not that many cards.
then for a pair, you just check if any two adjesent cards are of the same value
code: |
for (int i=1; i<=4; i++)
{
if card(i) == card(i+1)
//pair found
}
|
same for 3 of a kind and 4 of a kind.. just check more cards.
similarly for flush you check if ( card(n+1) == card(n)+1 ) for all cards.
|
|
|
|
|
 |
wtd
|
Posted: Wed Jan 05, 2005 9:55 pm Post subject: (No subject) |
|
|
Each card should be an object with two instance variables: suit and face.
code: | class Suit
{
public static final int DIAMOND = 1,
HEART = 2,
CLUB = 3,
SPADE = 4;
}
class Face
{
public static final int TWO = 1,
THREE = 2,
FOUR = 3,
FIVE = 4,
/* yada yada yada */;
}
class Card
{
private int suit, face;
public Card(int suit, int face)
{
this.suit = suit;
this.face = face;
}
public boolean equals(Card c)
{
// yada yada
}
public int compareTo(Card c)
{
// yada yada
}
public int getFace()
{
// yada yada
}
public int getSuit()
{
// yada yada
}
} |
Then you could create a card like:
code: | Card myCard = new Card(Suit.SPADE, Face.ACE); |
|
|
|
|
|
 |
leu_raz

|
Posted: Thu Jan 06, 2005 10:24 am Post subject: (No subject) |
|
|
thanks a lot.
I will start programming it and when i'm done i'm gonna post it so all of you could enjoy it. thx a lot.
|
|
|
|
|
 |
leu_raz

|
Posted: Wed Jan 12, 2005 6:33 pm Post subject: (No subject) |
|
|
tony wrote: the trick is to sort the cards in order by their face value. I don't remember if Java has any build in sorting algorythms, it shouldn't matter... not that many cards.
then for a pair, you just check if any two adjesent cards are of the same value
code: |
for (int i=1; i<=4; i++)
{
if card(i) == card(i+1)
//pair found
}
|
same for 3 of a kind and 4 of a kind.. just check more cards.
this seems very usefull to me. the thing is that i am importing pictures rite? so...i have to randomize the pictures(which is a pain in the behind) and also after that to know what did the user have and also how much he won or lost...i still need some more help.
P.S.: Tomoro i will post a version of my game and you'll see how far i've went with it. THX a lot compsci team
similarly for flush you check if ( card(n+1) == card(n)+1 ) for all cards.
|
|
|
|
|
 |
Andy
|
Posted: Wed Jan 12, 2005 7:21 pm Post subject: (No subject) |
|
|
leu_raz wrote:
similarly for flush you check if ( card(n+1) == card(n)+1 ) for all cards.
i dunno where ur getting that from but its not rite... all u need is a function called check flush, and pass down the hand object, and then with in it, just check hand.card(n).suit down the list
|
|
|
|
|
 |
Hikaru79
|
Posted: Wed Jan 12, 2005 10:08 pm Post subject: (No subject) |
|
|
leu_raz wrote:
this seems very usefull to me. the thing is that i am importing pictures rite? so...i have to randomize the pictures(which is a pain in the behind) and also after that to know what did the user have and also how much he won or lost...i still need some more help.
Getting a picture for each card shouldn't be hard. Just make the image be a part of the Card class and have the card assigned an image in it's constructor, once it recieves it's FACE and SUIT. Then it should just be a simple task of deciding which picture to give it based on it's stats, and then a simple method that returns the image that belongs to the card to be drawn.
Let me know if you need some psuedo code for that. Or better yet, post what you have so far. Easier that way
|
|
|
|
|
 |
leu_raz

|
Posted: Thu Jan 13, 2005 10:55 am Post subject: (No subject) |
|
|
So here is what i have so far. It's not finished cuz i didn't save what i did yesterday . My Bad...so look and see my code..........
P.S. : you need the whole folder for it....
Description: |
|
 Download |
Filename: |
Poker.java |
Filesize: |
3.47 KB |
Downloaded: |
730 Time(s) |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
leu_raz

|
Posted: Thu Jan 13, 2005 11:04 am Post subject: (No subject) |
|
|
Here...you'll need this too...........
Description: |
This is so that you a load a pic in only one line of code |
|
 Download |
Filename: |
GfxLib.java |
Filesize: |
1011 Bytes |
Downloaded: |
183 Time(s) |
|
|
|
|
|
 |
|